- Difference between checked and unchecked exceptions in java.
- Discuss the exception class hierarchy showing checked & unchecked exception in java.
- Discuss the examples of checked & unchecked exception.
- What is exception ?
- Exception is an event that occurs during the execution of program, the program breaks it normal flow of execution.
Types of exceptions in java
1.) Checked exception:
The exceptions which are checked by compiler (at compile time) are called checked exceptions. The compiler will reports the compilation error if the checked exceptions are not handled in program or method.The program can handle the exception using following options.
- catch the exception using try-catch block
- throw the exception from method.
Its common myth that checked exception is compile time exception, as discussed exception will occurs only during the execution of program. Compiler just forces to handle the checked exceptions during compilation process. (if not handled by program).
Examples of Checked exceptions:
- ClassNotFoundException
- IOException
- CloneNotSupportedException
- IllegalAccessException
- NoSuchFieldException
- NoSuchMethodException
- SQLException
- ParseException
Exception class hierarchy in java (shown some classes)
- The sub classes of Error and Runtime exception are called unchecked exceptions.
- The rest of the exceptions (except RuntimeException and Error sub classes) are called checked exceptions.
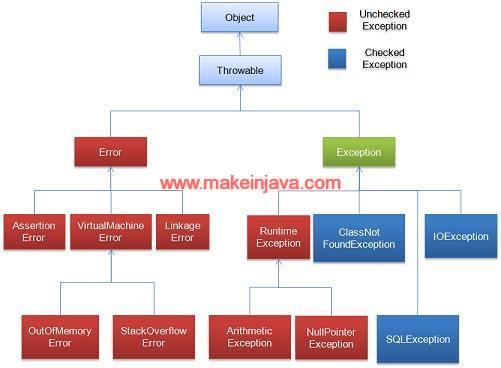
Program – Simulate checked exceptions in java
package org.learn; public class CheckExceptionDemo { public static void main(String[] args) { Class<?> objClass = Class.forName("java.lang.Integer"); System.out.println("Simple Name: " + objClass.getSimpleName()); System.out.println("Class Name: " + objClass.getName()); System.out.println("Class Package: " + objClass.getPackage()); } }
The above code will result in compilation error, either method main should catch the exception using try-catch block or method should throws the exception.
Exception in thread "main" java.lang.Error: Unresolved compilation problem: Unhandled exception type ClassNotFoundException at org.learn.CheckExceptionDemo.main(CheckExceptionDemo.java:5)
Catching checked exception using try catch block
package org.learn; public class CheckExceptionDemo { public static void main(String[] args) { Class<?> objClass; try { objClass = Class.forName("java.lang.Integer"); System.out.println("Simple Name: " + objClass.getSimpleName()); System.out.println("Class Name: " + objClass.getName()); System.out.println("Class Package: " + objClass.getPackage()); } catch (ClassNotFoundException e) { e.printStackTrace(); } } }
Program output – Catching ClassNotFoundException:
Simple Name: Integer Class Name: java.lang.Integer Class Package: package java.lang, Java Platform API Specification, version 1.8
2.) Unchecked exception:
The unchecked exceptions are not forced by compiler to implement. Unchecked exceptions are not checked at compiled time. Its purely at programmer’s discretion to catch or throws the unchecked exceptions.
Examples of Unchecked exceptions:
- ArithmeticException
- NullPointerException
- SecurityException
- ClassCastException
- IndexOutOfBoundsException
- OutOfMemoryError
- StackOverflowError
Program – Simulate unchecked exceptions in java
package org.learn; public class UnCheckedExceptionDemo { public static void main(String[] args) { catchArithmeticException(); } private static void catchArithmeticException() { int value = 15; int divideByZero = value / 0; System.out.println("Result of division by zero is: "+ divideByZero); } }
As compiler does not force to implements the unchecked exceptions, We need to anticipate possible occurrence of exception. We have shown the catchArithmeticException method, which does not catch the exception, so when we execute the above program, we will get following message.
Exception in thread "main" java.lang.ArithmeticException: / by zero at org.learn.UnCheckedExceptionDemo.catchArithmeticException(UnCheckedExceptionDemo.java:9) at org.learn.UnCheckedExceptionDemo.main(UnCheckedExceptionDemo.java:5)
Catching unchecked exception using try catch block,
package org.learn; public class UnCheckedExceptionDemo { public static void main(String[] args) { catchArithmeticException(); } private static void catchArithmeticException() { try { int value = 15; int divideByZero = value / 0; System.out.println("Result of divide by zero is: "+ divideByZero); } catch(ArithmeticException arithmeticException) { System.out.println("Exception occurred is:"+arithmeticException.getLocalizedMessage()); } } }
Program output – after catching ClassNotFoundException:
Exception occurred is:/ by zero